How to Automate PostgreSQL Trigger to Slack message
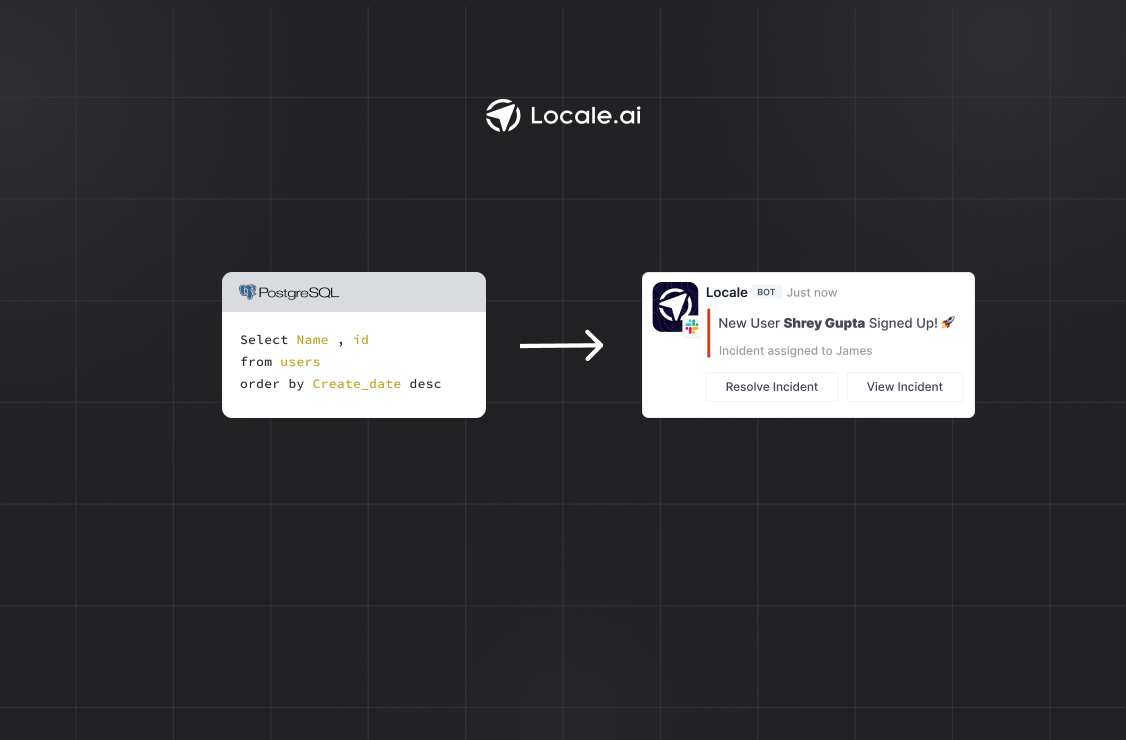
Automating PostgreSQL triggers to Slack can be a valuable way to keep your team informed of important events in your database. These triggers can help business simplify their process and make them more efficient. Timely notifications to Slack can help teams quickly respond to changes or issues. Suppose you're managing an e-commerce platform and want to get Slack notifications for every new order above a certain value. This real-time alerting could be essential for high-priority customer engagement or inventory management.
In this detailed guide, we’ll walk you through how to automate Postgres triggers to send Slack messages:
- Method #1: Using PostgreSQL NOTIFY/LISTEN with Python
- Method #2: Automating Trigger through Locale
Method #1: Using PostgreSQL NOTIFY/LISTEN with Python
When dealing with real-time event notifications in PostgreSQL, NOTIFY and LISTEN commands can be quite powerful. In this method, the NOTIFY clause announces a message whenever a significant event occurs, and the LISTEN clause receives the messages and acts upon them. Let's see how we can configure them:
Setting up a Slack Webhook URL
Before you can start sending notifications to Slack, you need to set up an incoming webhook:
- Go to your Slack workspace's App Directory.
- Search for and select Incoming WebHooks.
- Click Add to Slack to create a new webhook.
- Choose the channel to which you want the notifications to be posted.
- Click the Add Incoming WebHooks integration button.
- Copy the Webhook URL provided — you'll use this in your Python script to send notifications to Slack.

Remember to keep your webhook URL secure; it's a key that allows messages to be sent to your Slack workspace.
Setting up the PostgreSQL trigger
Next, we set up a trigger in PostgreSQL. This trigger will NOTIFY a channel whenever a new order that meets our criteria is inserted into the orders table:
In this SQL snippet, row_to_json(NEW) converts the newly inserted row into a JSON format, making it easy to extract data points in our Python script. This is a very handy JSON function provided by PostgreSQL, you can read up more about it here.
When the trigger fires, the NEW record is transformed into JSON, encapsulating all the column values of the new order. This JSON is sent as a string payload with the NOTIFY command.
Sending the Slack message
Next, we need a Python script that listens for these notifications and sends a message to a Slack channel using a webhook. In this script, we are first setting up a connection our PostgreSQL server that will listen for incoming NOTIFY payloads of the function high_value_orders . Once we receive the payload, we use its data to create a Slack message body and send it using the Slack Webhook URL we previously obtained.
With this setup, when a new high-value order is placed, the trigger sends a notification, the Python script catches it, and a detailed Slack message is posted. It's a neat solution for real-time alerts, but it requires continuous script running and careful handling of the webhook URL security. It comes with a set of limitations which can make the whole process complex for you:
- This becomes less efficient as the scale of data increases leading to bottlenecks in high-traffic databases.
- Implementing and maintaining triggers for multiple tables and operations can be complex and error-prone, and requires constant monitoring.
- NOTIFY does not support built-in filtering or aggregation of notifications. This means that listeners receive all messages leading to an overwhelming amount of noise.
- It relies on the Slack Webhook being correctly configured and secured.
Let’s look at the other method, which can help you avoid these issues and is easy to set up.
Method #2: Automating Trigger through Locale
Locale is a no-code platform which significantly simplifies the process of PostgreSQL query runs. Automating PostgreSQL query through locale is an easy deal, With just a few clicks you can automate the PostgreSQL query results to Slack. Let’s see how you can automate through Locale.
Step 1: Login to Locale, Go to Org Setting and Connect your Postgres database from the data source.
Step 2: Now go to Alerts → Create New Alerts. Choose your database → Click on Setup data to be monitored.
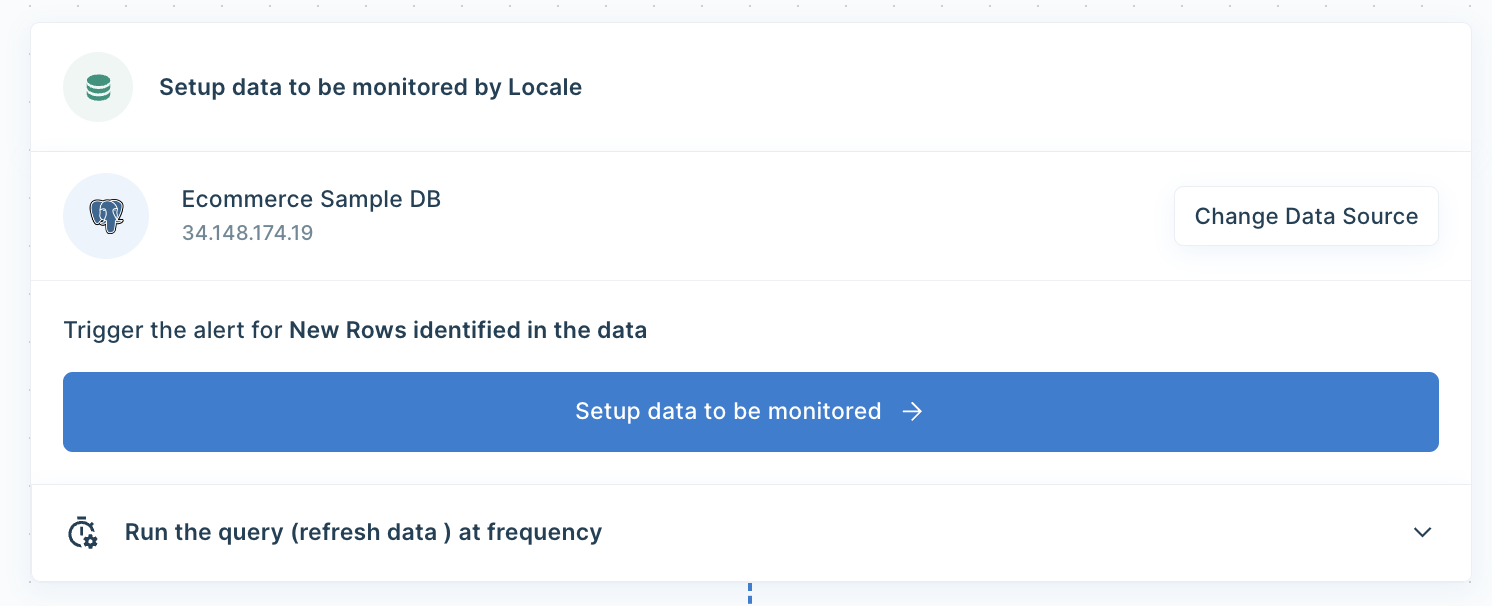
Step 3: Write your own SQL Query and click Run to verify the data. Once you are satisfied, click Done.

In the above example, if you want to get notified about large orders, you can use the below SQL script:
Step 4: Now Setup your schedule based on your requirement, this would automatically run the query based on the frequency you set. Click on Run the query at frequency and choose your schedule

Step 5: With Locale, you can also customize the Slack message to get the better idea. To configure the Slack message, Click on Configure incidents and enter your message in the Incident Title.

Step 6: Set up whom or on which channel the Slack alert should be sent. Go to the Setup Notification section and click on Add Notification then select Send Slack Message. Select whether you want the Slack message to be sent to a channel or a user. You can also preview and send test notifications.

Save the alert. It will automatically run itself on the pre-set schedule and trigger a Slack notification.
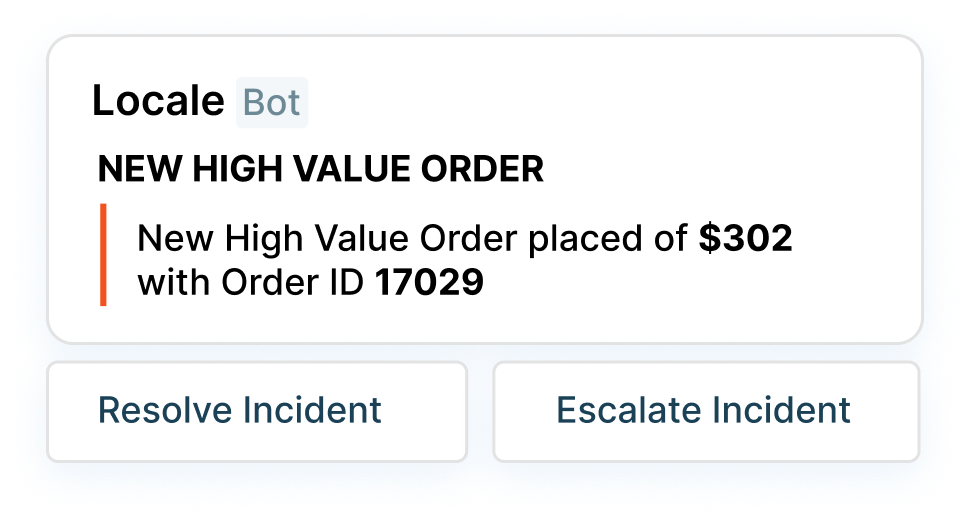
Conclusion
Automating PostgreSQL triggers to Slack helps your team to be notified on their preferred channel so that they can quickly response to changes or issues. In this detailed guide, we looked at two methods which can help you to automate Slack alerts for PostgreSQL query results.
However, while Method 1 (using PostgreSQL NOTIFY/LISTEN with Python) is a viable option, it can be complex and error-prone to implement, especially if you need to handle complex notification scenarios. Additionally, you need to keep your Python script up to date with any changes to your PostgreSQL database or Slack configuration.
Locale’s no-code platform makes it easy to automate PostgreSQL triggers to Slack with minimal one-time setup. Locale helps you to:
- Easy automation of PostgreSQL triggers to Slack
- Choose a schedule for sending notifications based on your requirements.
- Dynamically choose people to send Slack message based on the query result allowing alerts only go to the person it needs.
- Group the notifications ensuring less noise to the users.
- Set up and provide a clear playbook for users to follow in case of any issue so that it can be resolved efficiently.
- Set up an escalation rule to ensure issues are being resolved.
- Moreover, if you want to expand sending reports/data to other destinations like Gmail, WhatsApp, MS Teams etc, you get to do that with 100+ integration options.
If you are looking for a simple and reliable way to automate PostgreSQL triggers to Slack, Try Locale. It takes less than 10 minutes to get all things set up: Try for free