How to Automate Sending Slack Messages using Node.js

TL;DR
This blog explains two methods to automate sending Slack messages using Node.js, with example on fetching daily currency exchange rates.
Method #1: Using Node.js and ExchangeRatesAPI:
- Understand ExchangeRatesAPI and obtain an API key.
- Set up Slack webhook to receive notifications.
- Implement a Node.js script to fetch exchange rates and send Slack messages.
Method #2: Connect any API on Locale.ai for Slack alerts:
- Use Locale's API Connector to connect data sources through API.
- Create alerts in Locale and configure schedules for alert runs.
- Set up recipients and customize Slack messages.
Automating Slack messages with Node.js requires technical skills and may face scalability and maintenance challenges. However Locale.ai ‘s no-code platform offers a simpler solution to automate Slack messages from any API. It also offers dynamic notifications based on query results. This streamlines communication within teams without extensive development efforts.
In the modern workplace, regular updates and notifications are essential for keeping teams aligned and informed. One efficient way to achieve this is by automating Slack messages that carry important data from various APIs. This not only enhances productivity but also ensures that everyone is on the same page with the latest information.
In this blog, we'll demonstrate this concept using a practical example: sending automated Slack messages with daily currency exchange rates from the ExchangeRatesAPI. While we focus on currency data, this method can be adapted to various other APIs, making it a versatile solution for different business needs.
- Method #1: Using Node.js and ExchangeRatesAPI for Slack Notifications
- Method #2: Connect API on Locale and send Slack alert
Method #1: Using Node.js and ExchangeRatesAPI for Slack Notifications
Step 1: Understanding ExchangeRatesAPI
ExchangeRatesAPI provides real-time exchange rate data for over 200 currencies. It's a straightforward and accessible tool, ideal for our demonstration of fetching the USD to INR (US Dollar to Indian Rupee) exchange rate. The API is free for basic usage, which includes up to 1,000 monthly requests. To get started, sign up for their official website and obtain your API key.
Step 2: Setting Up a Slack Webhook
To automate Slack messages, the first step is to set up a Slack webhook:
- Go to your Slack workspace's App Directory.
- Search for and select Incoming WebHooks.
- Click Add to Slack to create a new webhook.
- Choose the channel to which you want the notifications to be posted.
- Click the Add Incoming WebHooks integration button.
- Copy the webhook URL provided — you'll use this in your Python script to send notifications to Slack.
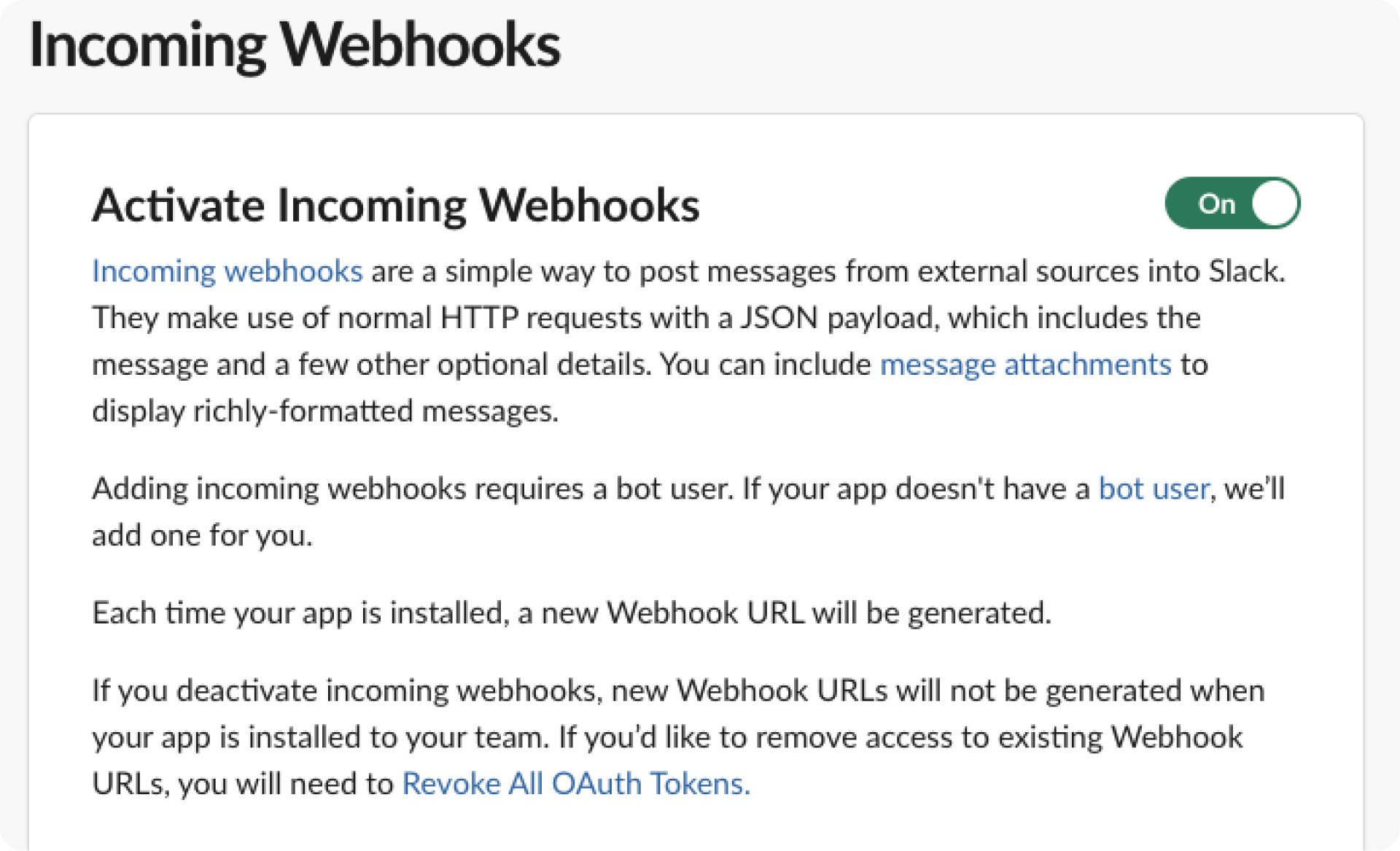
Step 3: Implementing the Node.js Script
With your API key and Slack webhook URL, you can now create a Node.js script to send daily currency updates:
This script fetches the latest USD to INR exchange rate from ExchangeRatesAPI and sends it as a Slack message.
Drawbacks
While effective, this method has its challenges:
- Technical Expertise Required: Setting up and maintaining the script requires knowledge of Node.js
- Complex Error Handling: Robust error handling is essential to manage potential API failures or network issues, adding complexity to the script.
- Limited Scalability: The script may need significant modifications for more complex requirements or higher data volumes, affecting scalability.
Method #2: Connect API on Locale and send Slack alert
Locale is a no-code platform which significantly simplifies the process sending Slack alert from any API. With just a few clicks, you can automate the Slack alerts using SQL queries.
Step 1: Login to Locale, go to Org Setting and select API Connector to connect your data source through API.
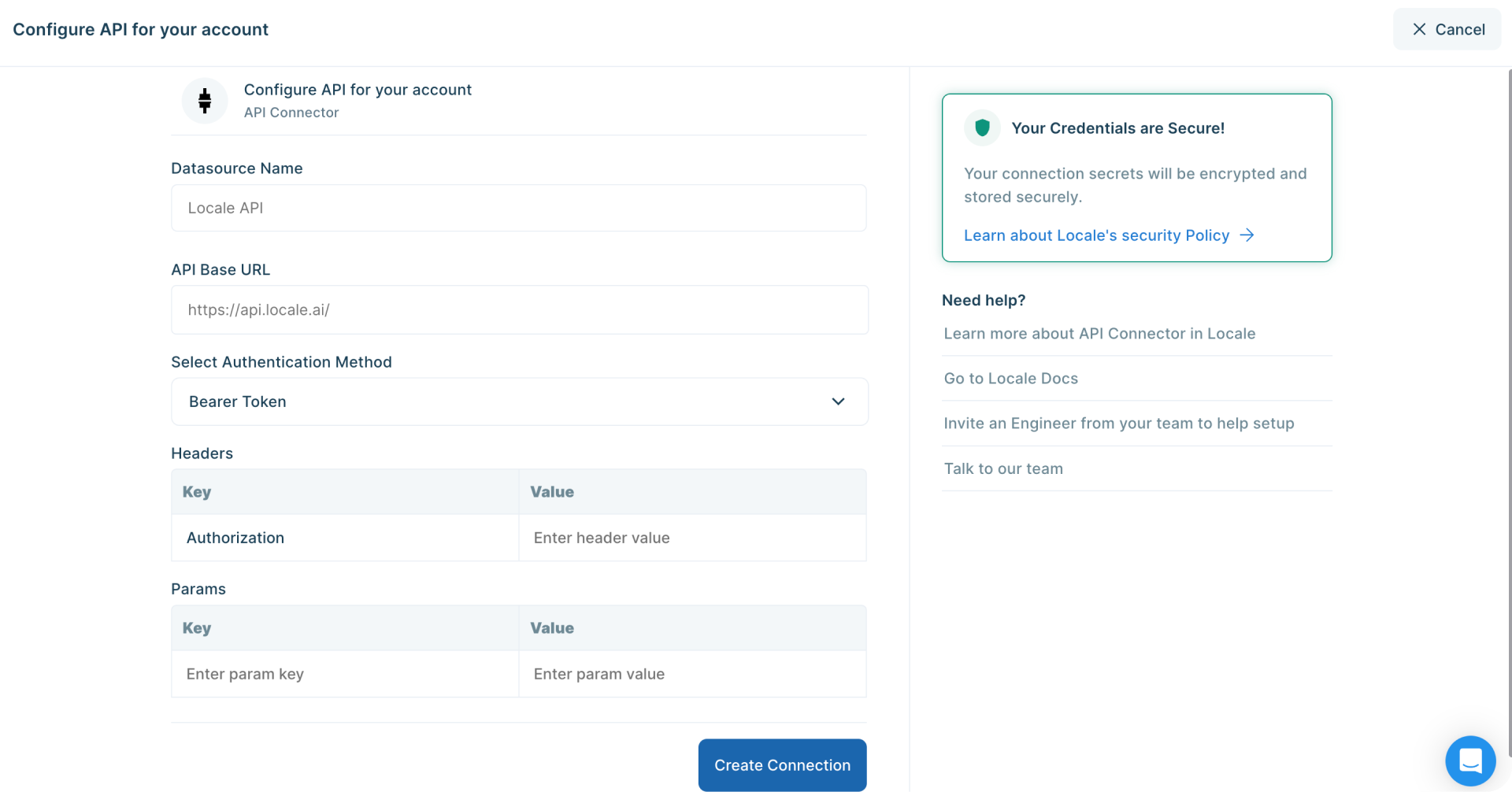
Step 2: Go to Alerts → Create New Alerts. Choose your database → Click on Setup data to be monitored. Add your API endpoint, write your query and then click on Run Query. Once, it fetches the correct data, click on Done.
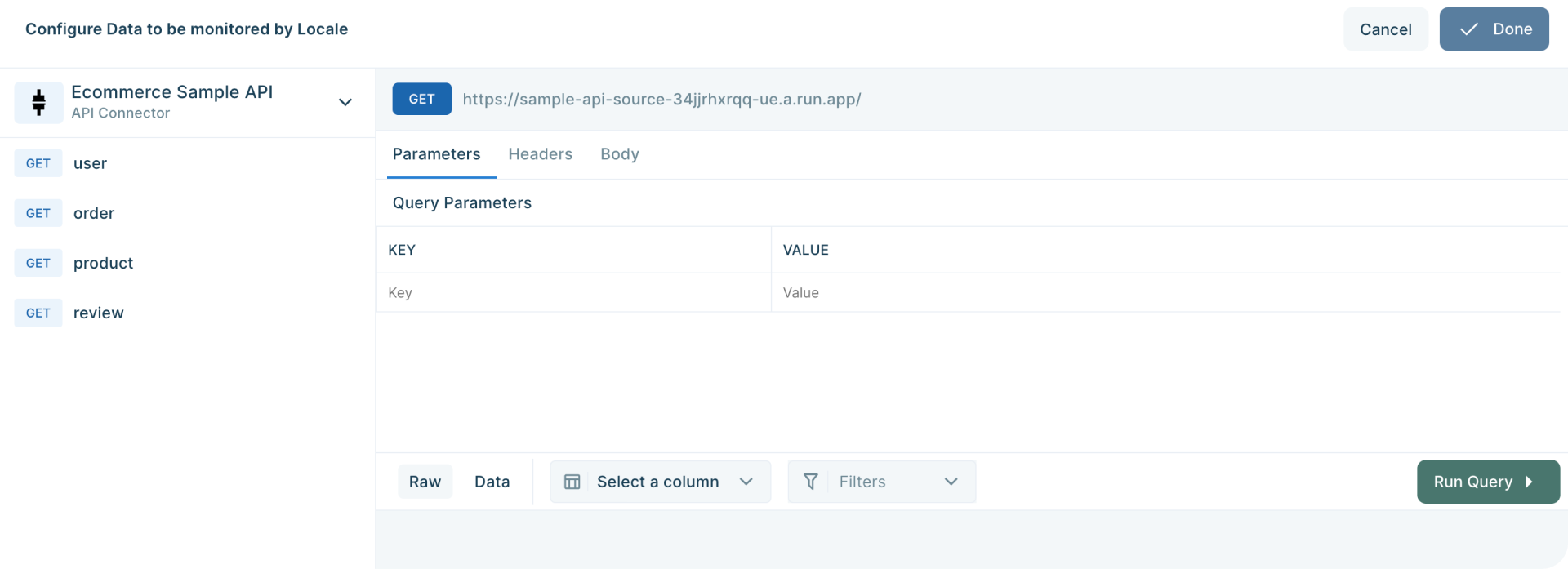
Step 4: Now Setup your schedule based on your requirement, this would automatically run the query based on the frequency you set. Click on Run the query at frequency and choose your schedule
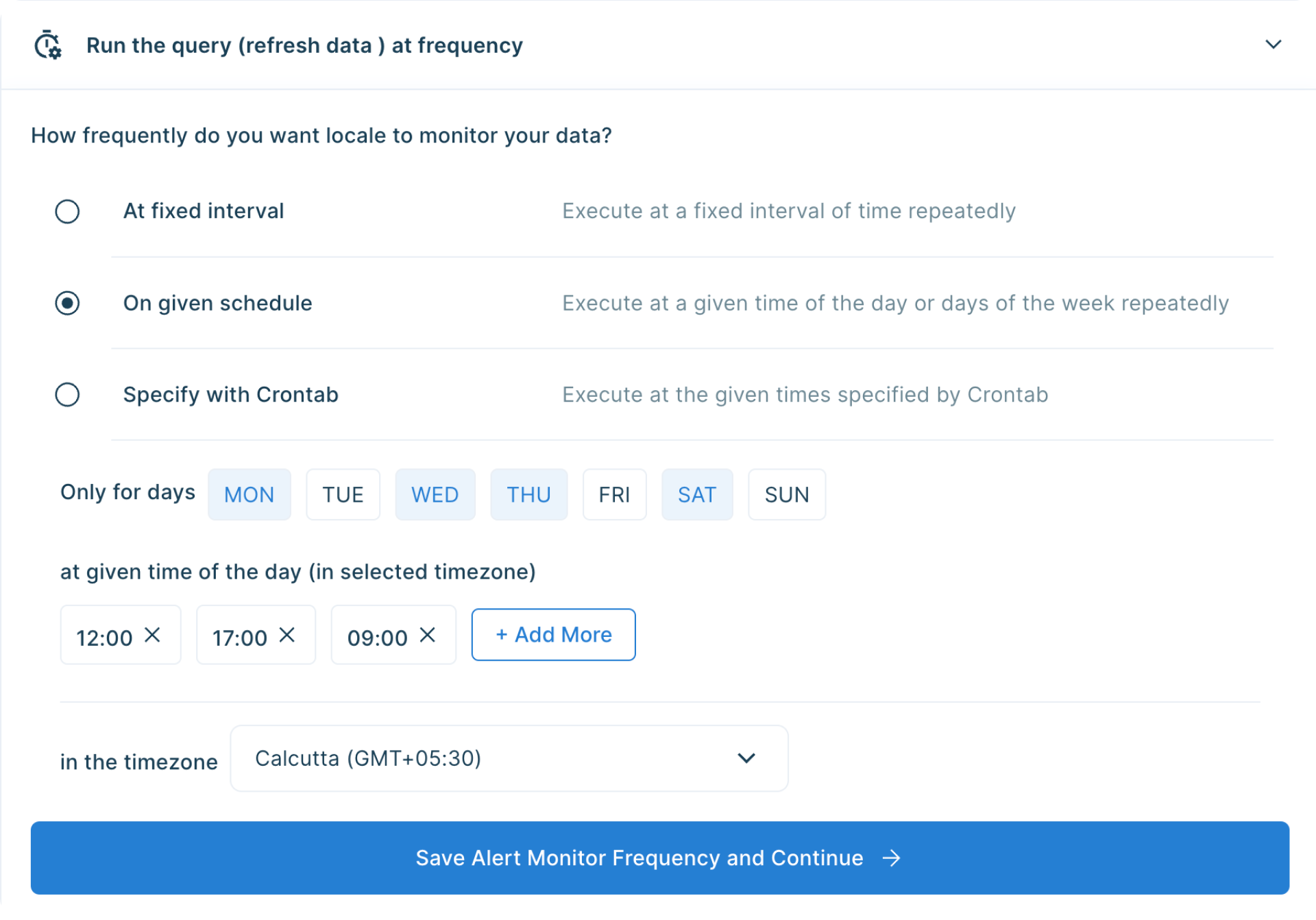
Step 5: With Locale, you can also customize the Slack message to get a better idea. To configure the Slack message, click on Configure Incidents and enter your message in the Incident Title.
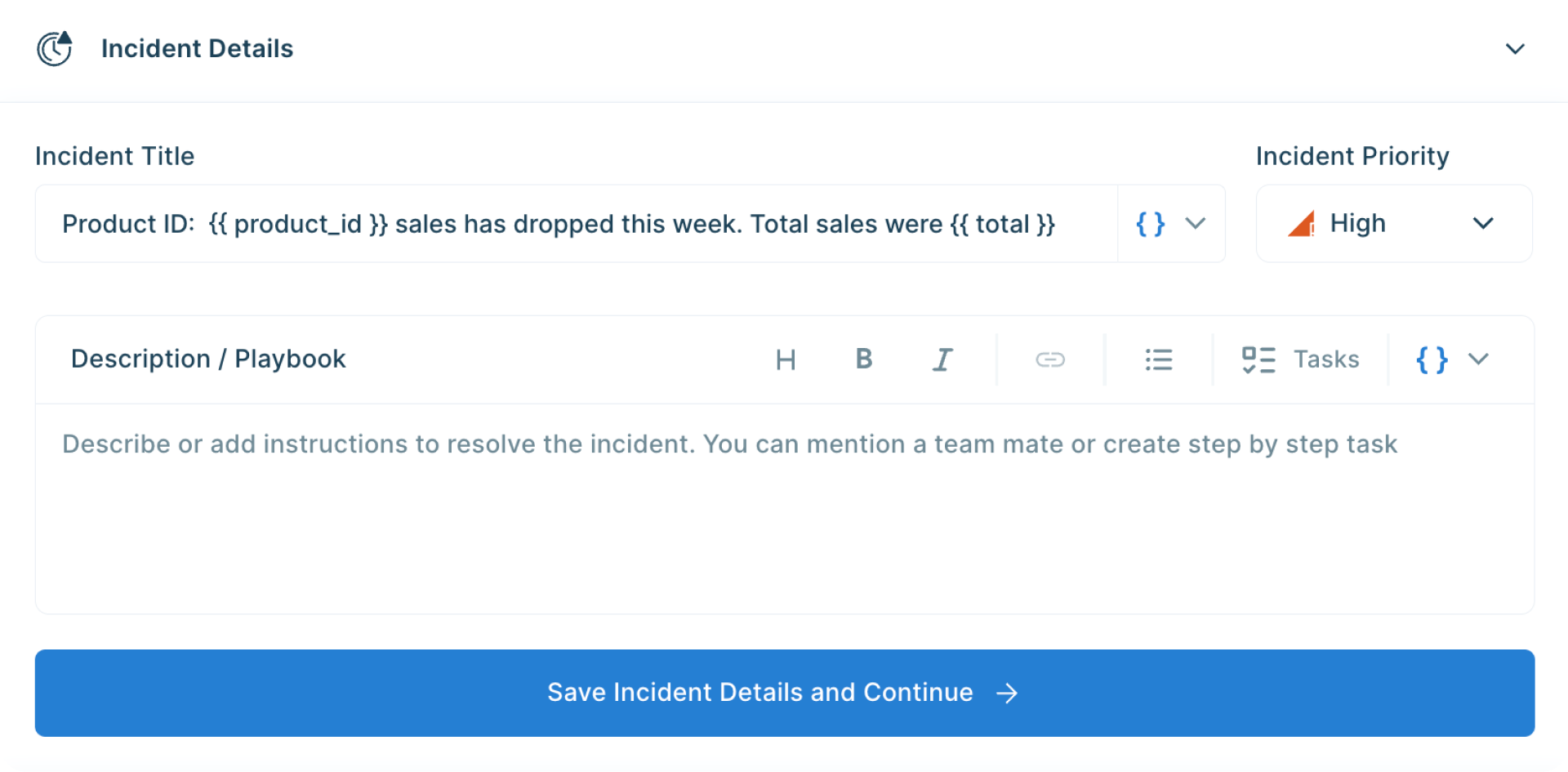
Step 6: Final Step, set up whom or on which channel the Slack alert should be sent. Go to the Setup Notification section and click on Add Notification then select Send Slack Message. Select whether you want the Slack message to be sent to a channel or a user. You can also preview and send test notifications.
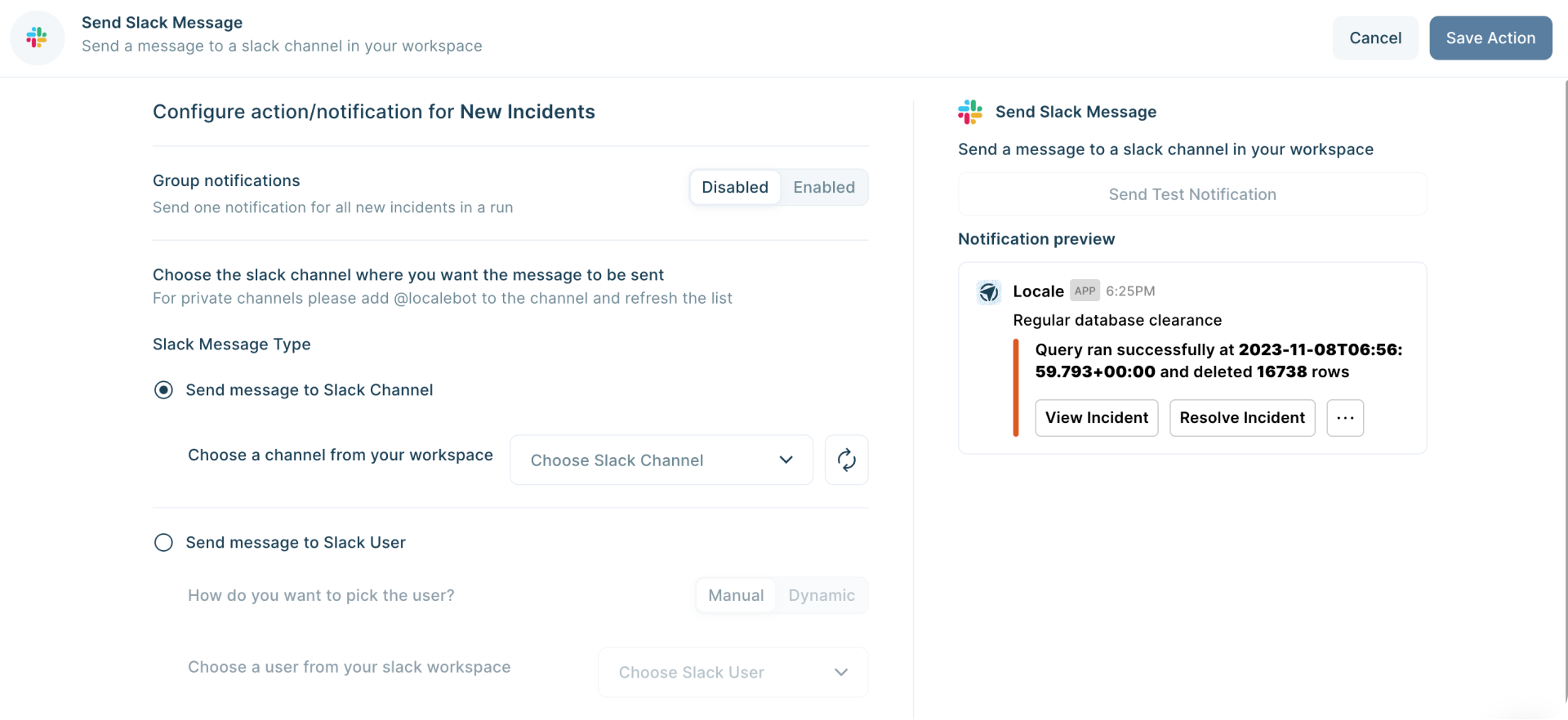
You can also dynamically send Slack messages to the users based on query results. To set up this, Select Send message to Slack User → Select Dynamic, then choose the field.
Save the alert. It will automatically run itself on the pre-set schedule and trigger a Slack notification.
Conclusion
Automating Slack messages with no-code platform like Locale offers valuable solutions for streamlining communication within teams. It’s no-code platform simplifies the entire process, allowing users to effortlessly connect APIs, automate Slack alerts, and customize notifications with just a few clicks. It provides users a hassle-free environment empowering users to automate Slack messages effortlessly, streamlining communication without the need for extensive development efforts. It also allows sending Slack messages dynamically to different users, help delivering important details only to those it’s neccessary and decluttering the noise.
Excited to get started? It takes less than 10 minutes to get all things set up: Try for free